First sorry about my bad english.
I animate via physics engine and render a bunch of cubes.
If the the center of a cube geomtry (center of bounding box) are out of the window (camera frustum) the complete cube are not visible.
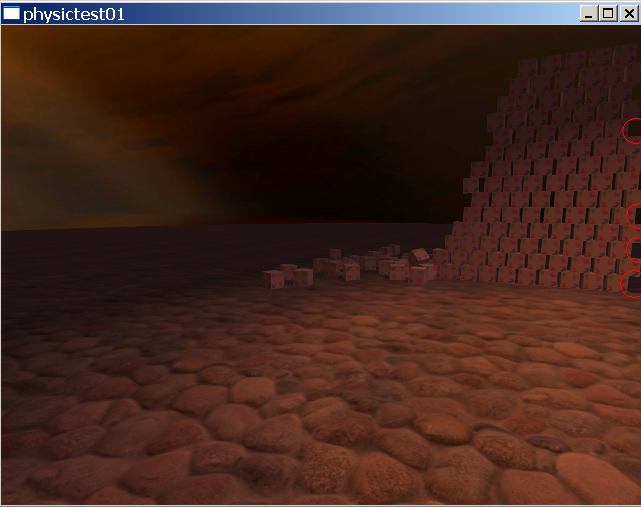
What i'm doing wrong ?
Thank you.
Joshy
Code:
#include once "fbgfx.bi"
#include once "horde3d.bi"
#include once "tokamak-c.bi"
type AnimatedBody as neAnimatedBody ptr
type RigidBody as neRigidBody ptr
' a static massless body with collision geometry
function CreateAnimatedBodyBox(byval sim as neSimulator ptr, _
byval fWidth as single=1, _
byval fHeight as single=1, _
byval fDepth as single=1) as neAnimatedBody ptr
var ab = SimulatorCreateAnimatedBody(sim)
var geo = AnimatedBodyAddGeometry(ab)
if fWidth <0.01 then fWidth =0.01
if fHeight<0.01 then fHeight=0.01
if fDepth <0.01 then fDepth =0.01
GeometrySetBoxSize(geo, fWidth,fHeight,fDepth)
AnimatedBodyUpdateBoundingInfo(ab)
return ab
end function
' a dynamic body with mass and collision geometry
function CreateRigidBodyBox(byval sim as neSimulator ptr, _
byval fWidth as single=1, _
byval fHeight as single=1, _
byval fDepth as single=1, _
byval fMass as single=1) as neRigidBody ptr
var rb = SimulatorCreateRigidBody(sim)
var geo = RigidBodyAddGeometry(rb)
if fWidth <0.01 then fWidth =0.01
if fHeight<0.01 then fHeight=0.01
if fDepth <0.01 then fDepth =0.01
if fMass <1 then fMass =1
GeometrySetBoxSize(geo, fWidth,fHeight,fDepth)
RigidBodySetInertiaTensor(rb,neBoxInertiaTensor(fWidth,fHeight,fDepth,fMass))
RigidBodySetMass(rb,fMass)
RigidBodyUpdateBoundingInfo(rb)
return rb
end function
function InitHorde3D(byval w as integer=640,byval h as integer=480) as boolean
dim as integer bits
screeninfo ,,bits
' create window with OpenGL context
screenres w,h,bits,,fb.GFX_OPENGL
if screenptr()=0 then
screen 0
print "error: init OpenGL context !"
beep : sleep : end 1
end if
flip
' init Horde3D
var ret = h3dInit()
if ret=false then h3dutDumpMessages()
return ret
end function
dim as integer iWidth=640,iHeight=480
'screeninfo iWidth,iHeight
'iWidth*=0.75 : iHeight*=0.75
if InitHorde3D(iWidth, iHeight)=false then
screen 0
print "error: h3dInit() !"
beep : sleep : end 1
end if
var sim = CreateSimulator()
if sim=NULL then
h3dRelease()
screen 0
print "error: CreateSimulator() !"
beep : sleep : end 1
end if
' optinal set dump filename and title
dim as string dumpFile = command(0) & ".html"
dim as string dumpTitle = "tokamak 1.0.5 game physics test with: " & *h3dGetVersionString
h3dutSetDumpFile (strptr(dumpFile))
h3dutSetDumpTitle(strptr(dumpTitle))
h3dutDumpMessages()
' Add resources
var renderPipeline = h3dAddPipeline ("pipelines/forward.pipeline.xml")
var skyBoxScene = h3dAddSceneGraph("models/skybox/skybox.scene.xml")
var platformScene = h3dAddSceneGraph("models/platform/platform.scene.xml")
var cubeScene = h3dAddSceneGraph("models/cube/cube.scene.xml")
' Load resources from content folder
var contentDir = exepath() + "/Content"
h3dutLoadResourcesFromDisk(strptr(contentDir))
' optional dump log messages
h3dutDumpMessages() ' usefull if loading of any resources are fails
var skyboxNode = h3dAddNodes(H3DRootNode, skyboxScene)
h3dSetNodeTransform(skyboxNode, 0,0,0, 0,0,0, 200,200,200)
h3dSetNodeFlags (skyboxNode, H3DNodeFlags_NoCastShadow, true )
var platformNode = h3dAddNodes(H3DRootNode, platformScene)
var platformBody = CreateAnimatedBodyBox(sim,150,2,150)
AnimatedBodySetPosition(platformBody,0,-1,0)
const MAX_CUBES = DEFAULT_RIGIDBODIES_COUNT
const SQR_CUBES = sqr(MAX_CUBES)
dim as TH3DNode cubeNode(MAX_CUBES-1)
dim as RigidBody cubeBody(MAX_CUBES-1)
for i as integer = 0 to MAX_CUBES-1
cubeNode(i) = h3dAddNodes(H3DRootNode, cubeScene)
cubeBody(i) = CreateRigidBodyBox(sim)
var col = i \ SQR_CUBES
var row = i mod SQR_CUBES
RigidBodySetPosition(cubeBody(i),-SQR_CUBES\2 + col*1.2 + (i and 1)*0.5,.5+row,0)
next
var cam = h3dAddCameraNode(H3DRootNode, "Camera", RenderPipeline)
h3dSetNodeTransform(cam, 0,5,30, -10,0,0, 1,1,1)
' Setup position and size of the viewport
h3dSetNodeParamI(cam, H3DCamera_ViewportXI , 0)
h3dSetNodeParamI(cam, H3DCamera_ViewportYI , 0)
h3dSetNodeParamI(cam, H3DCamera_ViewportWidthI , iWidth)
h3dSetNodeParamI(cam, H3DCamera_ViewportHeightI, iHeight)
' Set camera parameters (field of view, asspect ratio, near plane, far plane)
h3dSetupCameraView( cam, 60.0, iWidth / iHeight, 0.1, 1000.0 )
' Setup size of the render target
h3dResizePipelineBuffers(RenderPipeline, iWidth, iHeight)
' Add a light
var light = h3dAddLightNode(H3DRootNode, "Light1", 0, "LIGHTING", "SHADOWMAP")
h3dSetNodeTransform(light, 5,25,15, -90,0,0, 1,1,1)
h3dSetNodeParamF(light, H3DLight_RadiusF , 0, 50)
h3dSetNodeParamF(light, H3DLight_FovF , 0, 90)
h3dSetNodeParamI(light, H3DLight_ShadowMapCountI, 1)
h3dSetNodeParamF(light, H3DLight_ShadowMapBiasF ,0, 0.01)
h3dSetNodeParamF(light, H3DLight_ColorF3, 0, 0.8) ' red
h3dSetNodeParamF(light, H3DLight_ColorF3, 1, 0.4) ' green
h3dSetNodeParamF(light, H3DLight_ColorF3, 2, 0.2) ' blue
h3dutDumpMessages()
' shows the bounding boxes
'h3dSetOption(H3DOptions_DebugViewMode,1)
' usefull if something are wrong with trriangles
'h3dSetOption(H3DOptions_WireframeMode,1)
dim as single xPos,yPos,zPos
dim as single xRot,yRot,zRot
dim as single matrix(15)
dim as single ptr transform = @matrix(0)
const CAM_TARGET = 10
while inkey()=""
SimulatorAdvance(sim)
RigidBodyGetOpenGLMatrix(cubeBody(CAM_TARGET),transform)
h3dutLookAt(cam,0,5,30, transform[12], transform[13], transform[14], 0,1,0)
for i as integer = 0 to MAX_CUBES-1
RigidBodyGetOpenGLMatrix(cubeBody(i),transform)
h3dSetNodeTransMat (cubeNode(i),transform)
next
' Render scene
h3dRender( cam )
' Finish rendering of frame
h3dFinalizeFrame()
' swap buffers
flip
wend
' free all resources
h3dRelease()
DestroySimulator(sim)